How to create custom layout in android
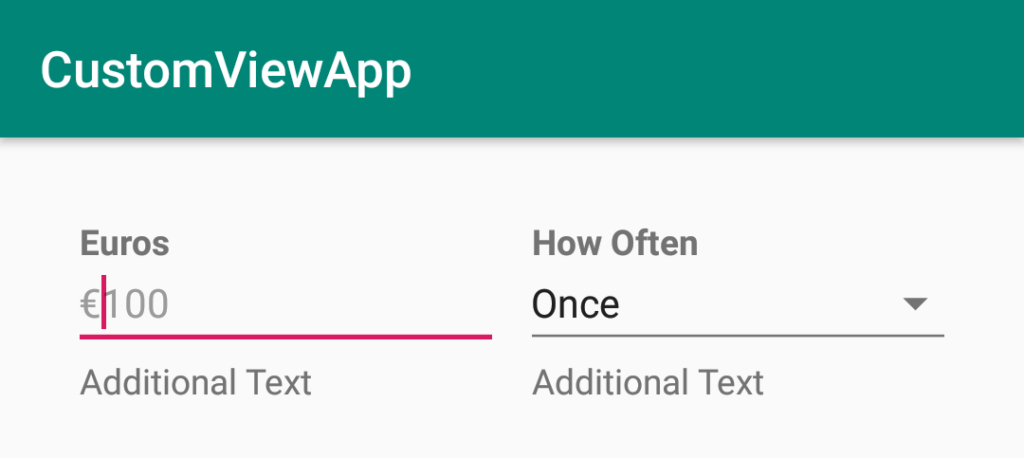
Creating a custom layout in Android allows developers to tailor the user interface to specific needs and preferences. This process involves understanding the fundamentals of Android layouts, utilizing XML for design, and potentially writing custom view components in Java or Kotlin. Whether you're aiming to improve the user experience, add complex functionality, or simply bring a unique aesthetic to your app, mastering custom layouts is an essential skill for any aspiring Android developer. In this article, we'll guide you through the steps to create your own custom layout and bring your creative vision to life in your Android applications.
How to create a custom layout in Android?
Creating a custom layout in Android allows developers to define a unique structure and behavior for the user interface of an application. To create a custom layout, you can either extend an existing layout class or create one from scratch by extending the `ViewGroup` class. Below are the steps to create a custom layout:
1. Choose a Base Class: Decide if you want to extend an existing layout (like LinearLayout, RelativeLayout, etc.) or extend the `ViewGroup` class for more control.
2. Create a New Java Class: Create a new Java class that extends your chosen base class. For example:
```java
public class CustomLayout extends ViewGroup {
// Constructor
public CustomLayout(Context context) {
super(context);
}
// Additional constructors as needed
}
```
3. Override Required Methods: At minimum, you should override the following methods:
- `onMeasure(int widthMeasureSpec, int heightMeasureSpec)`: Measure the layout's children.
- `onLayout(boolean changed, int l, int t, int r, int b)`: Position the children within the layout.
4. Customize Measurement: In `onMeasure`, calculate the size requirements for your layout and each of its children, then call `setMeasuredDimension(int width, int height)` with the desired size.
5. Customize Layout: In `onLayout`, assign a position and size to each child by calling `layout(int left, int top, int right, int bottom)` on the children.
6. Handle Attributes: If you want to allow customization through XML, define custom attributes in `res/values/attrs.xml` and handle them in your class constructor.
7. Create XML Layout (Optional): You can define your custom layout in XML, similar to how you would use built-in layouts:
```xml
```
8. Use in Activity or Fragment: Instantiate your custom layout in code or use it in an XML layout file, then set it as the content view or add it to your view hierarchy.
Remember to handle view state persistence and accessibility if necessary. Developing a custom layout requires a good understanding of how Android's view measurement and layout process works, so make sure to consult the official Android documentation for more detailed information and best practices.
How do I create a custom layout?
Creating a custom layout usually involves a combination of HTML, CSS, and potentially JavaScript or other programming languages, depending on the complexity and functionality of the layout you want to create. Here are the general steps you should follow:
1. Define the structure of your layout using HTML. You'll want to use semantic elements where appropriate, such as `
How to create a layout File in Android?
Creating a layout file in Android is a fundamental step in designing the user interface of an application. Layout files are usually written in XML and define the visual structure for your UI. Here's how you can create a layout file:
1. Open Android Studio: Start by launching Android Studio, the official integrated development environment (IDE) for Android development.
2. Navigate to the Project Window: In the Android view, navigate to the 'app' directory, and then further to the 'res' directory, followed by the 'layout' directory.
3. Create a New Layout File:
- Right-click on the 'layout' directory.
- Go to 'New' > 'Layout resource file'.
- A dialog window will appear prompting you to name your layout file.
4. Enter the File Name: Choose a name for your layout file. By convention, layout file names are written using underscores, e.g., `activity_main.xml` or `fragment_detail.xml`.
5. Choose the Root Element: You will also have the option to select a root element for your layout, such as `LinearLayout`, `RelativeLayout`, `ConstraintLayout`, etc. This will be the parent view that contains all other views within your layout.
6. Click 'OK': After naming your file and choosing the root element, click 'OK' to create the file.
7. Edit the Layout XML: The new file will open in the editor, and you can start adding UI elements. Each element in the layout is defined with XML tags, and you can set properties such as `layout_width`, `layout_height`, `layout_gravity`, and many others to control the appearance and behavior of the views.
8. Add Views: Drag and drop different widgets from the Palette into your layout, or write the XML manually to add elements like `TextView`, `Button`, `ImageView`, etc.
9. Save the File: Once you have finished designing your layout, save the file. Android Studio automatically compiles the XML layout file and generates a corresponding binary file in the `res` directory.
10. Preview the Layout: Use the Preview pane in Android Studio to see how your layout would appear on different devices and configurations.
Remember to always keep in mind the best practices of Android UI design, such as responsive layouts that adapt to different screen sizes and densities, and accessibility for users with disabilities. Good layout design is key to creating an intuitive and user-friendly application.
How to design Android app layout?
Designing an Android app layout is a crucial step in creating a user-friendly application. The layout determines how the app's content is organized and presented to the user. Here are the steps and best practices for designing an Android app layout:
1. Understand the User Requirements: Before starting the design process, it's essential to understand the target audience and their requirements. This includes researching user behavior, preferences, and the problems the app intends to solve.
2. Choose a Layout Type: Android provides several layout types such as LinearLayout, RelativeLayout, FrameLayout, and ConstraintLayout. Choose the one that best suits your app's design needs.
- LinearLayout arranges elements in a single direction, either horizontally or vertically.
- RelativeLayout allows positioning elements relative to each other or to the parent container.
- FrameLayout is designed to block out an area on the screen to display a single item.
- ConstraintLayout is a more flexible and advanced layout that allows for flat view hierarchies and complex layouts using constraints.
3. Use XML for Layout Definition: Android app layouts are typically defined in XML files. This allows separation of layout and view styling from the app's business logic, which is usually written in Java or Kotlin.
4. Utilize the Design Editor: Android Studio provides a Design Editor that offers a drag-and-drop interface for designing layouts. It is a valuable tool for quickly assembling layouts and previewing how they will look on various devices and screen sizes.
5. Consider Multiple Screens: Android devices come in various screen sizes and densities. Design your layout using density-independent pixels (dp) and scale-independent pixels (sp) for font sizes to ensure that your app provides a consistent user experience across all devices.
6. Implement Responsive Layouts: Use responsive design techniques such as size classes and breakpoints to make sure your app looks good on both phones and tablets. Test your layouts on different screen sizes and orientations.
7. Optimize for Performance: Keep your layout hierarchy as flat as possible. Nested layouts can lead to decreased performance. Where necessary, consider using the `
8. Accessibility: Design with accessibility in mind. Ensure that your app is usable by as many people as possible by following accessibility guidelines, such as providing content descriptions for UI elements and ensuring adequate contrast ratios.
9. Test Your Layout: Always test your layouts on actual devices or emulators to identify and fix any issues. Use the Android Profiler in Android Studio to monitor the performance of your app and make necessary optimizations.
10. Iterate Based on Feedback: After testing, collect user feedback and make iterative improvements to your app's layout. User testing can reveal insights that you may not have considered during the initial design phase.
By following these steps and best practices, you can create an Android app layout that is both aesthetically pleasing and functional, providing a great user experience. Remember to keep the user at the center of your design process and continuously iterate based on real-world use and feedback.
How to create custom layout in android xml
Creating a custom layout in Android XML allows you to define the user interface for your app with a high degree of control and flexibility. Here's how to create one:
1. **Start with a Layout File**
- Create a new XML file in the `res/layout` directory of your Android project. This file will define the structure of your custom layout.
2. **Define the Root Element**
- Choose a layout manager as the root element that will hold all other UI components. Common choices include `LinearLayout`, `RelativeLayout`, and `ConstraintLayout`. For example:
```xml
3. **Add UI Components**
- Inside the root element, add the UI components that you need, such as `TextView`, `Button`, `ImageView`, etc. Each component should have layout properties defined, such as `android:layout_width` and `android:layout_height`.
- For example, to add a TextView:
```xml
```
4. **Customize Attributes**
- You can customize each UI component by setting attributes like `android:textSize`, `android:layout_margin`, `android:drawableLeft`, and many others to adjust the appearance and layout behavior.
5. **Use @string and @dimen Resources**
- Instead of hardcoding text and dimensions, use resources from `strings.xml` and `dimens.xml`. This promotes reusability and simplifies localization and maintenance.
```xml
```
6. **Include Other Layouts**
- You can include other layout files within your custom layout to modularize your UI. Use the `
```xml
```
7. **Add Custom Views**
- For more advanced customization, you can create a custom view by extending an existing view class. You'll need to create a new Java or Kotlin class and override the necessary methods. Then, you can include your custom view in your XML layout using its fully qualified class name.
```xml
```
8. **Preview and Adjust**
- Use Android Studio's Layout Editor to preview your custom layout and make adjustments as needed. The editor provides a visual representation of your layout, and you can drag and drop UI components.
9. **Use in Your Activity or Fragment**
- Finally, use the custom layout in your `Activity` or `Fragment` by setting it as the content view or inflating it. For an `Activity`, use:
```java
setContentView(R.layout.my_custom_layout);
```
For a `Fragment`, use in the `onCreateView` method:
```java
View view = inflater.inflate(R.layout.my_custom_layout, container, false);
```
By following these steps, you can create a custom layout in Android XML that suits the specific needs of your application, providing a tailored user experience.
We leave you with one last piece of advice for having made it this far: Always ensure your custom layout is well-optimized for performance and accessibility, and test it across different devices and screen sizes for a seamless user experience.
Goodbye.




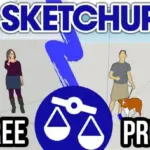
If you want to know more about similar articles like How to create custom layout in android you can visit category Landscaping Software.
Deja una respuesta